반응형
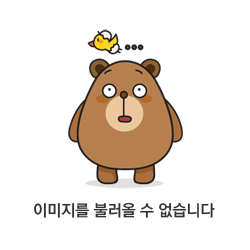
이 글의 동영상 강의입니다.
AppModule에서 설정했던 TypeORM 설정을 외부 파일로 분리하면 관리가 좀 더 편해집니다.
그리고 Entity 파일도 동적으로 읽어오게 하면 Entity가 생길때마다 추가해야하는 일을 줄일 수 있습니다.
위 2가지 방법으로 기존 소스를 변경해 보겠습니다.
기존 AppModule에 TypeOrm은 아래와 같이 설정하였습니다.
src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { CatsModule } from './cats/cats.module';
import { Cat } from './cats/entity/cats.entity';
import { AuthModule } from './auth/auth.module';
@Module({
imports: [
TypeOrmModule.forRoot({
type: 'mysql',
host: 'localhost',
port: 3306,
username: 'root',
password: 'root',
database: 'test',
entities: [Cat],
synchronize: true,
}),
CatsModule,
AuthModule
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
이를 다음과 같이 외부 설정 파일로 분리할 수 있습니다.
src/orm.config.ts
import { TypeOrmModuleOptions } from '@nestjs/typeorm';
function ormConfig(): TypeOrmModuleOptions {
const commonConf = {
SYNCRONIZE: false,
ENTITIES: [__dirname + '/domain/*.entity{.ts,.js}'],
MIGRATIONS: [__dirname + '/migrations/**/*{.ts,.js}'],
CLI: {
migrationsDir: 'src/migrations',
},
MIGRATIONS_RUN: false,
};
const ormconfig: TypeOrmModuleOptions = {
name: 'default',
type: 'mysql',
database: 'test',
host: 'localhost',
port: 13306,
username: 'root',
password: 'root',
logging: true,
synchronize: commonConf.SYNCRONIZE,
entities: commonConf.ENTITIES,
migrations: commonConf.MIGRATIONS,
cli: commonConf.CLI,
migrationsRun: commonConf.MIGRATIONS_RUN,
};
return ormconfig;
}
export { ormConfig };
Entity 파일들은 동적으로 읽어오기 위해 src/domain 폴더로 옮겨줍니다.
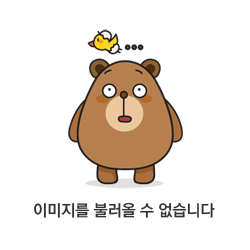
이렇게 설정 파일이 만들어지면 AppModule에서는 다음과 같이 변경하면 됩니다.
src/app.module.ts
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { CatsModule } from './cats/cats.module';
import { TypeOrmModule } from '@nestjs/typeorm';
import { AuthModule } from './auth/auth.module';
import { ormConfig } from './orm.config';
@Module({
imports: [
TypeOrmModule.forRootAsync({ useFactory: ormConfig }),
CatsModule,
AuthModule
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
이렇게 해서 좀 더 깔끔한 소스가 되었습니다.^^
반응형
'Nestjs 기초 동영상강좌' 카테고리의 다른 글
NestJS - 18. 설정 파일 & 환경 변수 관리 (4) | 2022.03.01 |
---|---|
NestJS - 17. 쿠키 다루기(JWT토큰) (0) | 2022.02.27 |
NestJS - 16. 파라메터 Null 체크 - ValidationPipe (0) | 2022.02.19 |
NestJS - 14. 권한 체크 (Role - Admin, User) (0) | 2022.02.02 |
NestJS - 13. JWT 토큰 인증 - Guard (2) | 2022.01.31 |
NestJS - 12. JWT 토큰 생성 (0) | 2022.01.30 |
NestJS - 11. 비밀번호 암호화(bcrypt) (2) | 2022.01.29 |
NestJS - 10. 로그인 #1 - 아이디/패스워드 체크 (0) | 2022.01.23 |